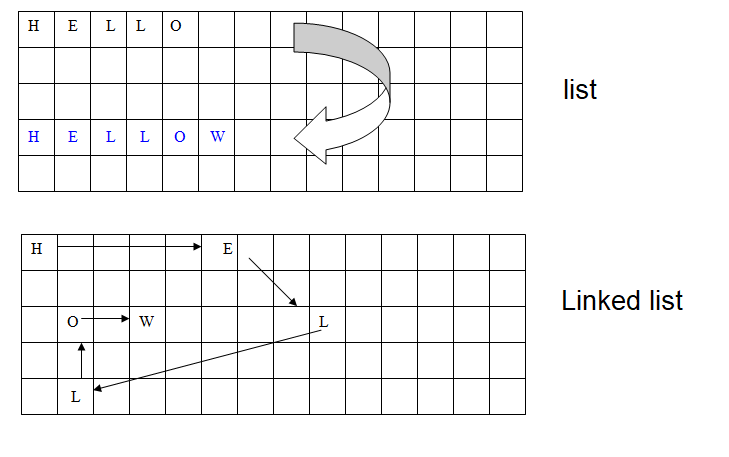
- What is dynamic memory allocation?
Dynamic memory allocation is a mechanism in programming languages like C and C++ that allows the allocation and deallocation of memory during program execution. It enables programs to allocate memory at runtime as needed, rather than statically allocating memory at compile time. - What are the most common memory allocation functions?
Memory Allocation Functions:
i.malloc(size_t size)
: Allocates a block of memory of the specified size in bytes and returns a pointer to the beginning of the allocated memory. The memory content is uninitialized.
ii.calloc(size_t num, size_t size)
: Allocates a block of memory for an array of elements, each of which has the size specified bysize
. It also initializes all bytes in the allocated memory to zero.
iii.realloc(void* ptr, size_t size)
: Changes the size of the memory block pointed to byptr
to the size specified bysize
. It may move the memory block to a new location. Ifptr
is NULL,realloc()
behaves likemalloc()
. Ifsize
is 0 andptr
is not NULL,realloc()
deallocates the memory block.
Memory Deallocation Function:
iv.free(void* ptr)
: Deallocates the memory block pointed to byptr
, making it available for further allocations. The pointerptr
must have been returned by a previous call tomalloc()
,calloc()
, orrealloc()
. - What is a linked list?
A linked list is a fundamental data structure consisting of a collection of nodes. Each node contains a piece of data and a reference (or pointer) to the next node in the sequence. Unlike arrays, which store elements in contiguous memory locations, linked lists utilize pointers to dynamically link nodes together, enabling efficient insertion and deletion operations. - How is a linked list different from an array?
Linked lists and arrays serve similar purposes of storing collections of data, but they differ in their underlying structure and behavior. Arrays offer direct access to elements through indexing, whereas linked lists require traversal from the head node to reach specific elements. Additionally, arrays have a fixed size determined at initialization, while linked lists can dynamically adjust their size by allocating memory as needed. - What are the basic components of a linked list?
The basic components of a linked list include nodes, which are the building blocks containing data and pointers/references. Each node typically comprises two parts: the data field, storing the actual element, and the next pointer, pointing to the next node in the sequence. - What is a node in a linked list?
A node is an elementary unit of a linked list, encapsulating a single data element and a reference to the next node in the sequence. It acts as a container that holds the data value and points to the following node, facilitating traversal and linkage within the list. - What is a self-referential structure, and how is it used in linked lists?
A self-referential structure, also known as a recursive structure, is a data structure in which an instance of the structure contains a reference to another instance of the same type. In linked lists, nodes are self-referential structures because each node contains a pointer to another node of the same type, enabling the formation of the linked structure. - How do you define a node struct in C/C++ for a singly linked list?
In C/C++, a node struct for a singly linked list is typically defined using a structure containing a data field and a pointer to the next node, as shown below:
struct Node {
int data;
struct Node* next;
};
9. Explain the concept of a “head” pointer in a linked list.
The “head” pointer in a linked list serves as the starting point or entry point for accessing the elements in the list. It points to the first node in the sequence, allowing traversal of the entire linked list by following the pointers from one node to another.
10. What is a null pointer and how is it used in linked lists?
A null pointer is a special value indicating that the pointer does not point to any valid memory address. In linked lists, null pointers are commonly used to denote the end of the list or the absence of a next node. When traversing the list, encountering a null pointer signifies reaching the end of the sequence.
11. What are the advantages and disadvantages of using linked lists compared to arrays?
Linked lists offer advantages such as dynamic memory allocation, efficient insertion and deletion operations (especially in the middle of the list), and flexibility in size adjustments. However, they also have disadvantages including additional memory overhead due to storing pointers, lack of direct access to elements (requiring sequential traversal), and potentially slower performance for certain operations compared to arrays.
12. How do you traverse a linked list?
Traversing a linked list involves iterating through each node in the sequence, starting from the head node and following the next pointers until reaching the end of the list (where the next pointer is null). This sequential traversal allows access to each element in the list for various operations.
13. How do you delete a node from a linked list?
Deleting a node from a linked list requires adjusting the pointers to bypass the node to be removed and deallocating its memory if necessary. This typically involves updating the next pointer of the preceding node to skip over the node to be deleted and freeing the memory occupied by the node.
14. What is the time complexity of various operations (insertion, deletion, searching) in a linked list?
- Insertion/Deletion at Beginning: O(1) time complexity since it involves updating only a constant number of pointers.
- Insertion/Deletion at End: O(n) time complexity due to the need to traverse the list to reach the insertion/deletion point.
- Searching: O(n) time complexity as it requires traversing the list sequentially until finding the desired element.
15. Explain the difference between singly linked lists and doubly linked lists.
- Singly Linked Lists: Each node in a singly linked list contains a reference to the next node in the sequence. Traversal can only occur in one direction (forward) from the head to the end of the list.
- Doubly Linked Lists: Nodes in a doubly linked list have references to both the next and previous nodes. This bidirectional linkage allows traversal in both forward and backward directions, enhancing flexibility but requiring additional memory for storing the backward pointers.
16. What is a circular linked list, and what are its advantages and disadvantages?
A circular linked list is a variant of a linked list where the last node points back to the first node, forming a circular structure. Advantages include efficient traversal from any point in the list and simplified handling of edge cases. However, disadvantages may include the potential for infinite loops if not properly managed and increased complexity in certain operations due to the circular structure.